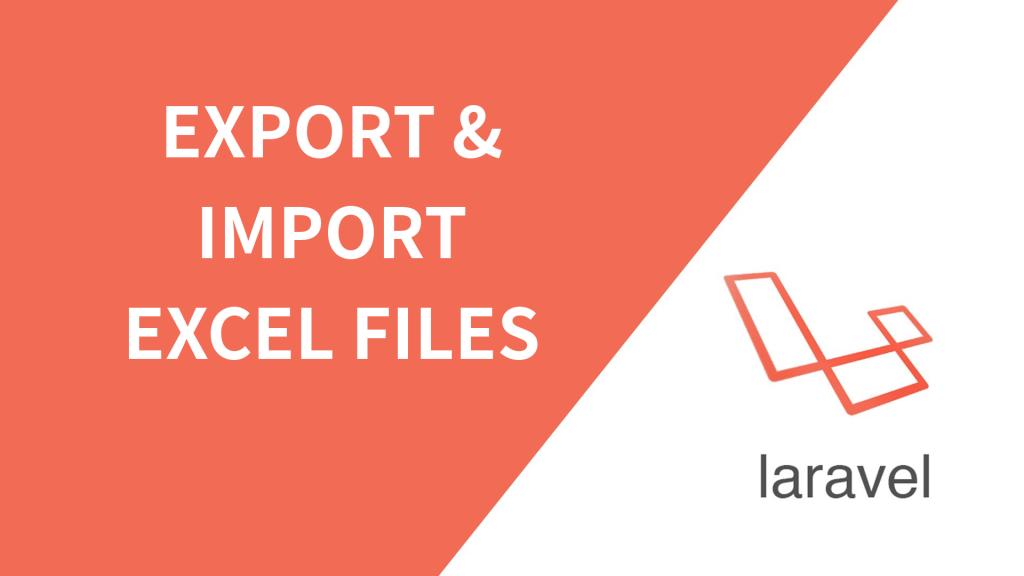
Laravel Excel được thiết kế như một phiên bản Laravel của PhpSpreadsheet. Đây là một class kế thừa và bổ sung cho PhpSpreadsheet để đơn giản hóa việc Export và Import dữ liệu từ các bảng tính. PhpSpreadsheet là một thư viện dựa trên PHP cho phép bạn đọc và ghi các định dạng tệp bảng tính khác nhau như Excel và LibreOffice Calc. Laravel Excel có những tính năng sau:
- Dễ dàng xuất các bộ sưu tập thành tệp Excel.
- Xuất các truy vấn với chia nhỏ tự động để tăng hiệu suất.
- Xếp hàng đợi để tăng hiệu suất.
- Dễ dàng xuất các giao diện Blade thành tệp Excel.
- Dễ dàng nhập dữ liệu vào các bộ sưu tập.
- Đọc tệp Excel theo từng phần.
- Xử lý việc chèn dữ liệu nhập khẩu theo lô.
Nếu bạn muốn tạo chức năng Export và Import tệp Excel dễ dàng, hướng dẫn này là ý hay cho bạn.
Yêu cầu
- PHP:
^7.2|^8.0
- Laravel:
^5.8
- PhpSpreadsheet:
^1.15
- PHP extension
php_zip
enabled - PHP extension
php_xml
enabled - PHP extension
php_gd2
enabled - PHP extension
php_iconv
enabled - PHP extension
php_simplexml
enabled - PHP extension
php_xmlreader
enabled - PHP extension
php_zlib
enabled
Bước 1: Cài đặt Dự án Laravel
Mở Terminal và chạy lệnh sau để tạo một dự án Laravel mới:
composer create-project --prefer-dist laravel/laravel laravel-excel
Hoặc, nếu bạn đã cài đặt Laravel Installer global composer:
laravel new laravel-excel
Bước 2: Cấu hình Chi tiết Cơ sở dữ liệu
Sau khi cài đặt xong, điều hướng đến thư mục gốc của dự án, mở tệp .env
và đặt chi tiết cơ sở dữ liệu như sau:
DB_CONNECTION=mysql DB_HOST=127.0.0.1 DB_PORT=3306 DB_DATABASE=<TÊN CƠ SỞ DỮ LIỆU> DB_USERNAME=<TÊN NGƯỜI DÙNG CƠ SỞ DỮ LIỆU> DB_PASSWORD=<MẬT KHẨU CƠ SỞ DỮ LIỆU>
Bước 3: Cài đặt gói maatwebsite/excel
Cài đặt Laravel Excel qua composer bằng lệnh sau:
composer require maatwebsite/excel
config/app.php
và thêm đoạn mã sau:'providers' => [ Maatwebsite\Excel\ExcelServiceProvider::class, ], 'aliases' => [ 'Excel' => Maatwebsite\Excel\Facades\Excel::class, ],
Sau đó, thực thi lệnh để export cấu hình:
php artisan vendor:publish --provider="Maatwebsite\Excel\ExcelServiceProvider" --tag=config
config/excel.php
.Bước 4: Tạo dữ liệu giả và di chuyển bảng
Trong Bước 1, chúng ta sẽ di chuyển bảng users
. Sau khi migration thành công, chúng ta sẽ tiếp tục Bước 2.
php artisan migrate
Trong Bước 2, chúng ta sẽ tạo dữ liệu giả. Sử dụng tinker
để tạo dữ liệu giả trong cơ sở dữ liệu:
php artisan tinker
Trong tinker
, chạy lệnh sau để tạo 100 bản ghi giả:
User::factory()->count(100)->create();
Bước 5: Tạo Route
Trong bước này, chúng ta sẽ thêm các route để xử lý yêu cầu export và import file
use App\Http\Controllers\UserController; Route::get('/file-import',[UserController::class,'importView'])->name('import-view'); Route::post('/import',[UserController::class,'import'])->name('import'); Route::get('/export-users',[UserController::class,'exportUsers'])->name('export-users');
Bước 6: Tạo Lớp Import
Maatwebsite cung cấp một lệnh để tạo class Import và chúng ta sẽ sử dụng nó trong controller. Chạy lệnh sau để tạo class import và sửa code trong tệp tạo ra:
php artisan make:import ImportUser --model=User
app/Imports/ImportUser.php
<?php namespace App\Imports; use App\Models\User; use Maatwebsite\Excel\Concerns\ToModel; class ImportUser implements ToModel { /** * @param array $row * * @return \Illuminate\Database\Eloquent\Model|null */ public function model(array $row) { return new User([ 'name' => $row[0], 'email' => $row[1], 'password' => bcrypt($row[2]), ]); } }
Ở đây, bạn có thể thấy cách ánh xạ giá trị cột CSV hoặc Excel vào Model Eloquent. Hãy định dạng cột CSV hoặc Excel theo cách bạn ánh xạ trong lớp nhập khẩu.
Bước 7: Tạo Lớp Export
Maatwebsite cung cấp một lênh Artisan để tạo class export và chúng ta sẽ sử dụng nó trong controller. Chạy lệnh sau để tạo class export và sửa code trong tệp tạo ra:
php artisan make:export ExportUser --model=User
app/Exports/ExportUser.php
<?php namespace App\Exports; use App\Models\User; use Maatwebsite\Excel\Concerns\FromCollection; class ExportUser implements FromCollection { /** * @return \Illuminate\Support\Collection */ public function collection() { return User::select('name', 'email')->get(); } }
Bước 8: Tạo Controller
Tiếp theo, chúng ta cần tạo một controller để hiển thị form tải lên tệp CSV hoặc Excel. Chạy lệnh sau để tạo controller và đặt code trong tệp tạo ra:
php artisan make:controller UserController
app/Http/Controllers/UserController.php
<?php namespace App\Http\Controllers; use Illuminate\Http\Request; use Maatwebsite\Excel\Facades\Excel; use App\Imports\ImportUser; use App\Exports\ExportUser; use App\Models\User; class UserController extends Controller { public function importView(Request $request) { return view('importFile'); } public function import(Request $request) { Excel::import(new ImportUser, $request->file('file')->store('files')); return redirect()->back(); } public function exportUsers(Request $request) { return Excel::download(new ExportUser, 'users.xlsx'); } }
Bước 9: Tạo Blade / View Files
Chúng ta đã đến bước cuối cùng. Ở đây, chúng ta cần tạo giao diện để xử lý Import và Export thông qua UI. Tạo tệp resources/views/importFile.blade.php
và đặt code sau trong tệp đó:
<!DOCTYPE html> <html lang="{{ str_replace('_', '-', app()->getLocale()) }}"> <head> <meta charset="utf-8"> <meta name="viewport" content="width=device-width, initial-scale=1"> <title>Laravel 8 Import Export Excel & CSV File - TechvBlogs</title> <link rel="stylesheet" href="https://stackpath.bootstrapcdn.com/bootstrap/4.5.0/css/bootstrap.min.css"> </head> <body> <div class="container mt-5 text-center"> <h2 class="mb-4"> Laravel 8 Import Export Excel & CSV File - <a href="https://techvblogs.com/blog/laravel-import-export-excel-csv-file?ref=repo" target="_blank">TechvBlogs</a> </h2> <form action="{{ route('import') }}" method="POST" enctype="multipart/form-data"> @csrf <div class="form-group mb-4"> <div class="custom-file text-left"> <input type="file" name="file" class="custom-file-input" id="customFile"> <label class="custom-file-label" for="customFile">Choose file</label> </div> </div> <button class="btn btn-primary">Import Users</button> <a class="btn btn-success" href="{{ route('export-users') }}">Export Users</a> </form> </div> </body> </html>
Chạy Ứng dụng Laravel
Cuối cùng, chúng ta phải chạy ứng dụng Laravel. Mở cửa sổ Terminal và chạy lệnh sau:
php artisan serve
Sau khi thực thi lệnh này, truy cập vào địa chỉ http://localhost:8000/file-import trong trình duyệt của bạn.
Cảm ơn bạn đã đọc bài viết này.